Controllers in Practice
Now that you understand the general function of controllers in ASP.NET, let's take a look at the process for creating one in Visual Studio.
The Controllers folder of a Visual Studio project contains the controller classes used to manage responses and input. Remember that the “Controller” suffix is required.
Begin by creating a file for a controller such as “SuperController.cs.” Next, design code to specify views.
namespace MySoftware.Controllers { public class CarController : Controller { // Get all the cars in the garage public ActionResult Index(id) { // Point to the view with content return View(cars); } } }
Note that Visual Studio allows code snippets to be saved and automatically generated; for example, on entering a term, a code template for a controller will be created.
ASP.NET MVC maps URLs to action methods within the Controllers class. The Views folder contains the information that defines the action result, which we will examine later. Note that controllers can contain as many methods as necessary to complete a task.
WRITING ACTION METHODS
Action methods are usually one-to-one. One-to-one refers to actions like entering a URL, clicking Submit, or clicking a link. They can return virtually any type of data, and though methods are diverse, they must conform to certain rules:
- Methods are public.
- Methods are not static.
- Methods are not extension methods.
- Methods are not constructors, getters, or setters.
- Methods do not contain open generic types.
- Methods are not methods of the controller base class.
- Methods do not contain ref or out parameters.
Review an example of an action method below:
public ActionResult Index() { var SelectedIndex = _rnd.Next(_cars.Count); ViewData["Cars"] = _cars[SelectedIndex]; return View(); }
WEB FORMS MODEL
ASP.NET web forms provide extension of the event-driven model into web applications causing a browser to send a web form to the server, which then returns a page with full markup. The server processes all client activities. ASP.NET works around HTTP's stateless nature by aiding in storing state information in its runtime.
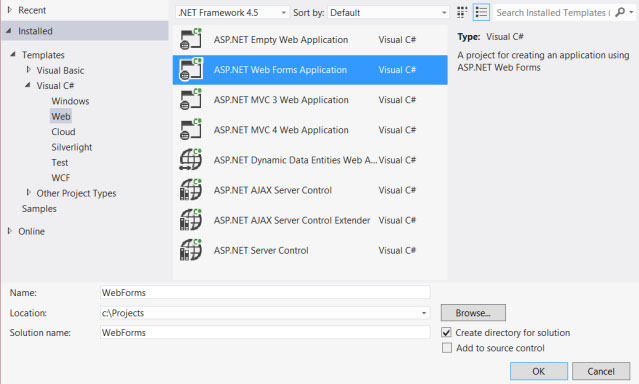
PARAMETERS
The default procedure for action method parameter values is to get them from data collected by the request. These value types include names or values from forms, query string values, and cookie values. If the value cannot be managed or if its type presents a problem, the passing of null or throwing of an exception occurs. The framework also automatically maps URL values to values for action methods through checking for HTTP request values by default on any incoming request.
There are many ways to access these values. Two means of access include the Request and Response objects of the Controller class. The Request object gathers values passed by the client to the server during an HTTP request. The Response object sends output to the client from the server. Review their syntax, and examples of their use below.
Correct syntax for Request follows:
Request[.collection|property|method](variable)
Correct syntax for Response follows:
Response.collection|property|method
Review examples of Request and Response code below:
strEmployeeID = Request.Form( "EmployeeID" )
Response.Clear
Response.Write "The number of strTheAmount is " & strTheAmount
Response.End