ASP.NET Core provides a built-in DI container that we can use to manage and inject dependencies. In ASP.NET Core, the DI container is configured in the "Startup.cs" file, making it easy to set up and use.
Basic ASP.NET Dependency Injection:
Let's start with a simple example. Suppose we have an interface "IMyLogger" and two implementations: "ConsoleLogger" and "FileLogger". We want to inject the appropriate logger implementation into our controller.
Define the IMyLogger Interface:
public interface IMyLogger
{
void Log(string message);
}
Implement the Logger Classes for ASP NET Dependency Injection:
public class ConsoleLogger : IMyLogger
{
public void Log(string message);
{
Console.WriteLine($"Logging to console: {message}");
}
}
public class FileLogger : IMyLogger
{
public void Log(string message)
{
// Log to a file
}
}
Configure ASP.NET Core Dependency Injection:
In your "Startup.cs" file, configure the DI container to use the "ConsoleLogger" implementation by default:
public void ConfigureServices(IServiceCollection services)
{
services.AddSingletonIMyLogger, ConsoleLogger>();
services.AddMvc();
}
In this code, we're using "AddSingleton", which means a single instance of "ConsoleLogger" will be shared among all requests.
Inject the Dependency into ASP.NET Core Controller:
Now, you can inject the "IMyLogger" dependency into your controller:
public class HomeController : Controller
{
private readonly IMyLogger _logger;
public HomeController(IMyLogger logger)
{
_logger = logger;
}
public IActionResult Index()
{
_logger.Log("Hello, ASP.NET Core!");
return View();
}
}
In the "HomeController", we inject the "IMyLogger" dependency through the constructor. Now, whenever the "Index" action is invoked, the appropriate logger implementation (in this case, "ConsoleLogger") will be used.
Constructor Injection, Method Injection,
and Property Injection
In the example above, we used constructor injection to inject dependencies. However, ASP.NET Core supports three common methods of injection:
- Constructor Injection: This is the recommended and most common method. It injects dependencies through the constructor, ensuring that required dependencies are available when the object is created.
- Method Injection: Dependencies can also be injected into methods when needed. This is useful for scenarios where you don't need the dependency for the entire object's lifetime.
- Property Injection: Dependencies can be injected into public properties of a class. While this is less common and generally discouraged due to its potential for misuse, it's still an option in some cases.
Here's a quick example of method injection:
public IActionResult Index([FromServices] IMyLogger logger)
{
logger.Log("Hello, ASP.NET Core!");
return View();
}
In this example, we use the "[FromServices]" attribute to inject the "IMyLogger" dependency into the "Index" action method. This method injection approach is particularly useful when you need a dependency for a specific action but not for the entire controller.
Lifetime of ASP.NET Core Dependencies
ASP.NET Core provides three options for controlling the lifetime of registered services:
- Transient: A new instance is created every time a service is requested. This is suitable for lightweight, stateless services.
- Scoped: A single instance is created and shared within the scope of a single HTTP request. It's appropriate for services that need to maintain state for
the duration of an HTTP request.
- Singleton: A single instance is created and shared across all requests. This is suitable for services that should be shared globally within the
application.
A single instance is created and shared across all requests. This is suitable for services that should be shared globally within the application.
services.AddTransient<IMyService, MyService>(); // Transient
services.AddScoped<IMyService, MyService>(); // Scoped
services.AddSingleton<IMyService, MyService>(); // Singleton
Advanced DOT NET Core Dependency Injection
So far, we've covered the basics of ASP.NET Core Dependency Injection. However, you can encounter more complex scenarios in real-world applications. Let's explore some advanced topics:
Conditional Registration
Sometimes, you may want to conditionally register a service based on certain criteria. For example, you might want to use a different service implementation depending on the environment (development, production, etc.). You can achieve this by conditionally adding services in your "Startup.cs" file:
if (env.
IsDevelopment())
{
services.AddTransient<IMyService, DevMyService>();
}
else
{
services.AddTransient<IMyService, ProdMyService>();
}
In this example, we register different implementations of "IMyService" based on the environment.
Working with Multiple Implementations
If you have multiple implementations of an interface and want to inject all of them, you can use enumeration injection. This allows you to work with all registered implementations as a collection:
public class MyController : Controller
{
private readonly IEnumerable _myServices;
public MyController(IEnumerable myServices)
{
_myServices = myServices;
}
// ...
}
In this example, "_myServices" will contain all registered implementations of "IMyService".
ASP NET Core Dependency Injection with Razor Views
In Razor views, you can use the "@inject" directive to inject services directly into the view. Here we are using ILogger interface of .NET, visit the .NET documentation page to read more about this.
@inject ILogger
<MyView
> Logger
<h1
>My View
</h1
>
<p
>Injecting logger
in a Razor view.
</p
>
@{
Logger.LogInformation("Logging from Razor view.");
}
This allows you to access services and data directly in your views.
Summary
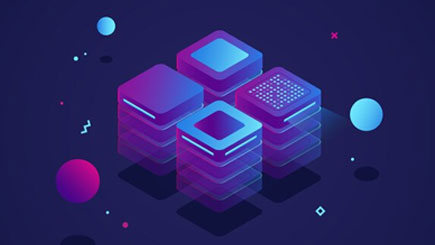
ASP.NET Core Dependency Injection is a powerful technique that promotes modular, maintainable, and testable code. It helps decouple components, making it easier to manage dependencies and write unit tests. In this article, we've covered the basics of Dependency Injection, including how to register and use services in ASP.NET Core, control their lifetimes, and handle more advanced scenarios.
By following the principles of Dependency Injection and the examples provided in this article, you'll be well-equipped to build robust and flexible ASP.NET Core applications. Whether you're a beginner or an experienced developer, embracing Dependency Injection can lead to more maintainable and scalable code in your ASP.NET Core projects.